Documents Generator API
Businesses create various documents from their enterprise systems and databases. The most common approach to automate this is by coding for each document template inside the core systems. This makes the code less modular and inflexible. There is minimal code reuse. Moreover, every time a template changes, the associated code requires modifications.
With EDocGen document generation API, business users can use existing templates as-is. They can create/edit PDF, Word, Excel, and PPTX templates in native editors without installing a separate designer or add-on. This reduces the dependency on the IT team for template changes. All the templates are stored in a central repository with a fine-grained authorization mechanism.
The enterprise document automation microservice helps the creation of documents from various data sources. It also offers pre-built integrations for document distribution. This makes it easy to create document workflows. The API automates document generation and distribution from enterprise applications and databases.
Document Automation Microservice
Developers can automate document generation with a few lines of code. They can create both on-demand and bulk documents from API.
- On-demand Generation - Creation through the synchronous API call. Faster creation and download of the file.
- Bulk Generation - The API is built for high performance and generates thousands of complex documents in a few seconds. Since these API calls are asynchronous, you can initiate high-volume requests.
You can generate almost all document formats, including Text, Image, Excel, PDF, PowerPoint, and Word. The REST API is flexible to support every document type and generation scenario across the enterprise.
Dynamic Field Types
It supports the dynamic population of text, tables, content blocks, hyperlinks, and images from Excel data. You can also include conditional statements, calculations, and loops right inside the template.
Text and tables Supports dynamic population of text, tables, loops, lists, and nested tables.
Images and QR Codes Populate Base64, Image URLs, QR Codes, and Barcodes dynamically.
HTML, Graphs & Charts The API allows you to populate HTML styles and charts.
Subtemplates, Paragraphs From a single master template, create variations by populating content blocks.
Apart from this, API also supports multi-lingual document templates, arithmetic calculations, and conditional statements (if-else) for generating proposals, invoices, contracts, etc.
Data sources
The microservice allows you to generate them from various data sources.
Data | JSON, XML, Excel |
Databases | MySQL, MSSQL, Oracle, MongoDB |
CRM | Salesforce, Dynamics 365 |
After generation, you can distribute generated documents through various channels including Email, e-Sign, print, and cloud storage.
E-Sign Providers | DocuSign, SignNow, Signaturely |
Document Management Systems (DMS) | SharePoint |
Cloud Storage | OneDrive, Box, Google Drive |
Email Providers | Amazon SES, SendGrid, Office365, GSuite |
Execution Steps
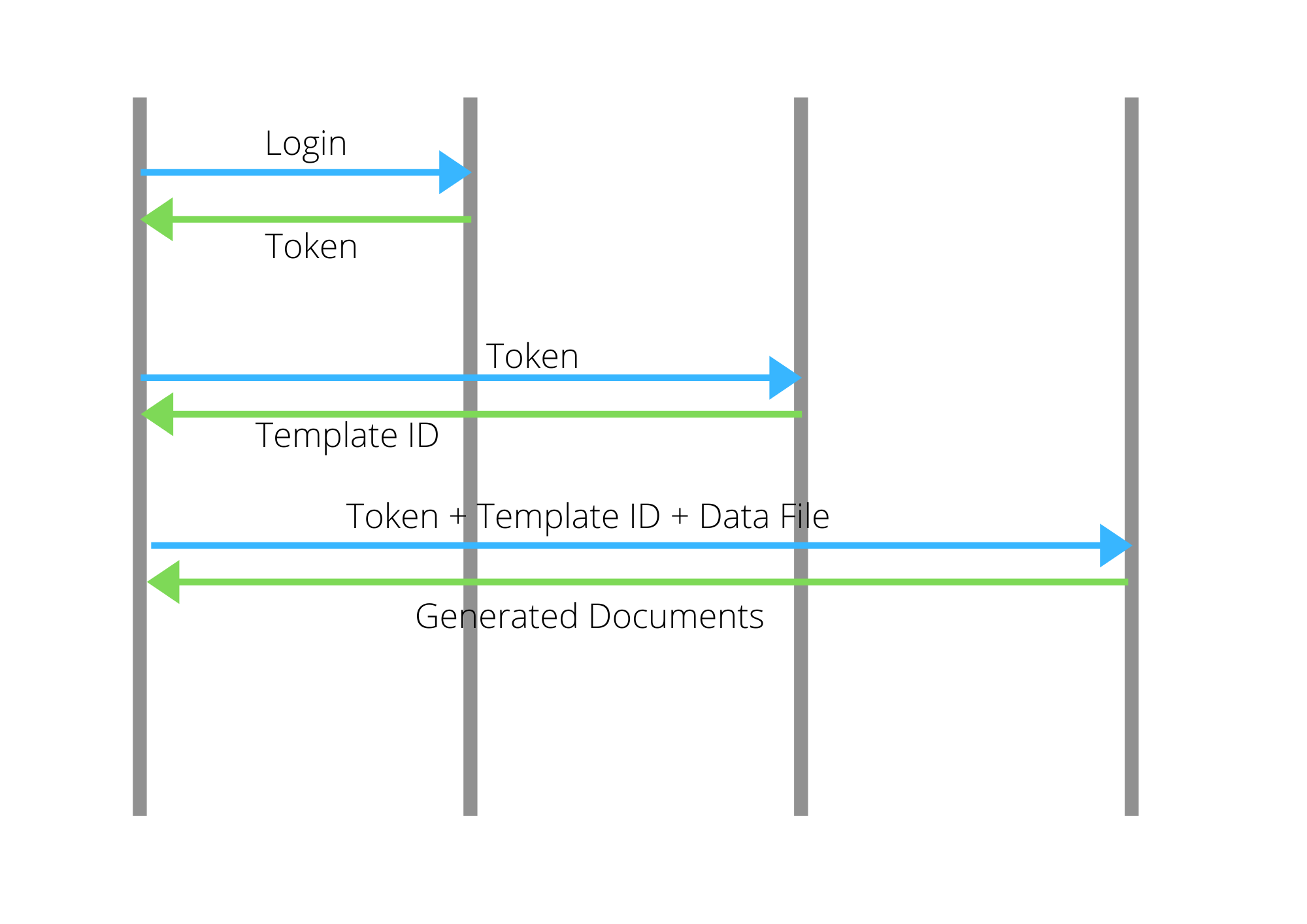
Here are the steps to authentication and making the REST API call to the EDocGen.
- API uses token-based web authentication. Register and obtain an access token by passing the username and password to /login. Use this token for every API call.
- The order of actions is Upload template -> Generate documents -> Search Output by name and get ID --> Download.
- Upload template through Post call.
- Get the template id.
$ curl 'https://app.edocgen.com/api/v1/document/?skipCount=15&limit=15&field=addedAt&sort_direction=-1&tags=none' -H 'User-Agent: Mozilla/5.0 (Macintosh; Intel Mac OS X 10.15; rv:86.0) Gecko/20100101 Firefox/86.0' -H 'Accept: application/json, text/plain, */*' -H 'Accept-Language: en-US,en;q=0.5' --compressed -H 'x-access-token: tokenid' | json_pp
{
"status" : 200,
"documents" : [
{
...
"_id" : "5f61dd26604d2322bb786d8e",
"name": "test.docx",
...
}
]
}
- Generate documents by passing template id and data file
curl 'https://app.edocgen.com/api/v1/document/generate/' -H 'User-Agent: Mozilla/5.0 (Macintosh; Intel Mac OS X 10.15; rv:86.0) Gecko/20100101 Firefox/86.0' -H 'Accept: application/json, text/plain, */*' -H 'Accept-Language: en-US,en;q=0.5' --compressed -H 'Content-Type: application/json;charset=utf-8' -H 'x-access-token: tokenid' -H 'Origin: https://app.edocgen.com' -H 'Connection: keep-alive' -H 'Referer: https://app.edocgen.com/template/' -H 'Cookie: continually_user_id=3eyk8pxzw6rn; _ga=GA1.2.1505829491.1596462685; x-session-id=s%3A4dezI-EfFmWK4ikHM9HirDfLq37ZHviq.mwKON5WAuvOQfBs8wF3tOgQYGSUhnd0KyhhgNWXQlAfbdg' --data-raw '{"markers":{"ATM":"1234567","MA":[{"SNo":"1","Local_Time":"12:00:01","Type":"Time","Currency":"$","Amount":"350","Cash_Amount":"300","Discount":"10","Profit":"50","Your_Share":"30"}],"Sum":"450"},"documentId":"5f61dd26604d2322bb786d8e","async":true,"format":"pdf","outputFileName":"Output.pdf"}'
Post data:
{
"markers": {
"ATM": "1234567",
"MA": [
{
"SNo": "1",
"Local_Time": "12:00:01",
"Type": "Time",
"Currency": "$",
"Amount": "350",
"Cash_Amount": "300",
"Discount": "10",
"Profit": "50",
"Your_Share": "30"
}
],
"Sum": "450"
},
"documentId": "5f61dd26604d2322bb786d8e",
"async": true,
"format": "pdf",
"outputFileName": "Output.pdf"
}
- Query for output files by name using /api/v1/output/name/{name}. Get the document identifier from the response. The file will only be available after a successful generation. To identify any problems with document generation, query events using /api/v1/events?startIndex=<startIndex>&endIndex=<endIndex>
- For downloading the output file, once the id and token are known, you can simply do a get to https://app.edocgen.com/api/v1/output/download/<id>?access_token=<access-token>. On clicking this URL, the browser automatically asks to save the output file. For bulk generation, the output is a zip file.
Programming Language Support
REST API can be consumed either as SAAS or can be deployed on on-premise servers to accommodate your specific performance and security needs. With the RESTful API, document generation becomes modular. Applications make a call to the API server, and the API returns the desired output.
Generate Office and PDF documents from practically any programming environment. It doesn’t matter what language and platform you are using because you make browser calls to API working in your preferred programming environment :
- JavaScript
- PHP
- Python
- Ruby
- Java
- C#
Java Python PHPJavaScript
package com.api.edocgen.service;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Bean;
import org.springframework.core.io.ByteArrayResource;
import org.springframework.http.*;
import org.springframework.stereotype.Component;
import org.springframework.stereotype.Service;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.web.client.HttpClientErrorException;
import org.springframework.web.client.RestTemplate;
import com.api.edocgen.dto.DBParameters;
import com.api.edocgen.dto.DocumentResponse;
import com.api.edocgen.dto.OutputDto;
import com.api.edocgen.dto.OutputResultDto;
import com.api.edocgen.util.Utils;
import java.io.File;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.Collections;
import java.util.UUID;
import javax.annotation.PostConstruct;
@Service
@Component
public class GenerateDocumentService {
@Autowired
private Utils utils;
@Autowired
RestTemplate restTemplate;
@Autowired
LoginService loginService;
/*
* Standard URLs
*/
public static String baseURL = "https://app.edocgen.com/api/v1/";
public static String urlBulkGenerate = "https://app.edocgen.com/api/v1/document/generate/bulk";
public static String urlOutputEmail = "https://app.edocgen.com/api/v1/output/email";
public static String urlQueryDocument = "https://app.edocgen.com/api/v1/document/?search_column=filename&search_value=";
/*
* User Configuration
*/
public static String outputFilePath = "./";
public static String pathFile = "JSON_Data.json";
public static String TemplateFileName = "edocgen_v1_union.docx";
@Bean
public RestTemplate restTemplate() {
return new RestTemplate();
}
private HttpHeaders headers;
@PostConstruct
public void postdata() throws Exception {
/*
*
* Database Configiuration Settings
*/
DBParameters dbParameters = new DBParameters();
dbParameters.setDbLimit("100");
dbParameters.setDbPassword("l8XuHH8Xax");
dbParameters.setDbVendor("mysql");
dbParameters.setDbUrl("jdbc:mysql://[email protected]:3306/sql6508014/sdtest");
dbParameters.setDbQuery("select * from sdtest");
/*
* This program supports following methods:
*
* ## Single File Generation API's ####
*
* getDocByApi(String documentId, String outputFileName, String outputFormat)
*
*
* ###### Bulk Files Generation API's #####
*
* getDocsByApi (TemplateId, OutputFormat) ====> This method generates bulk document based on JSON template which is present locally and downloads .zip file
* getDocsByDatabase(TemplateId , OutputFormat , DBConfiguration) ====> This method generates bulk document from database entries which is hosted in MySQL and downloads .zip file
*
* ## Generic Method works both for single and Bulk #####
* getTemplateId(TemplateFileName); ====> This method returns templateid based on filename
* sendOutputViaEmail(TemplateId , Email) ====> This method sends generated output files directly to email via given templateID.
*/
// String templateID = getTemplateId(TemplateFileName);
//getDocsByApi("62d95a226760033416374c10", "pdf");
getDocsByDatabase("62bdaf8308bcc4761b6aa6b9", "pdf", dbParameters);
// sendOutputViaEmail("62dfa645c2dae13e2ee6ec3e", "[email protected]");
// getDocByApi("62bdaf8308bcc4761b6aa6b9", "SDTest", "pdf");
}
public void getDocByApi(String documentId, String outputFileName, String outputFormat) throws Exception {
RestTemplate restTemplate = new RestTemplate();
try {
//convert file to byteArrayResource
ByteArrayResource resource = getByteArrayResource();
//create the body request
MultiValueMap<String, Object> body = createBody(resource,documentId,outputFileName,outputFormat);
//set Headers
headers = Utils.getHttpHeaders(MediaType.APPLICATION_JSON,
MediaType.MULTIPART_FORM_DATA, Utils.apiToken);
headers.set("x-access-token", utils.apiToken);
//send the request to generate document
HttpEntity requestEntity = new HttpEntity(body, headers);
ResponseEntity<String> generateResponse = restTemplate.postForEntity(urlBulkGenerate,
requestEntity, String.class);
String outputFile = outputFilePath + outputFileName + "." + outputFormat;
if (HttpStatus.OK == generateResponse.getStatusCode()) {
headers.clear();
headers.set("x-access-token", utils.apiToken);
requestEntity = new HttpEntity(null, headers);
ResponseEntity result = restTemplate.exchange(
baseURL + "output/name/" + outputFileName + "." + outputFormat , HttpMethod.GET,
requestEntity, OutputResultDto.class);
System.out.println("Send to edocGen Response : " + generateResponse.getStatusCode());
processOutput(result, outputFileName, outputFormat, requestEntity , true);
}
//concat to outputPath the name of the file and the format
//save the file
} catch (Exception e) {
System.out.print("Single File Generation Failed");
System.out.println(e.getMessage());
}
}
public String getTemplateId(String TemplateFileName) {
String resourceUrl = urlQueryDocument
+ TemplateFileName;
HttpHeaders headers = new HttpHeaders();
headers.set("x-access-token", utils.apiToken);
HttpEntity request = new HttpEntity(headers);
// Fetch JSON response as String wrapped in ResponseEntity
ResponseEntity response = restTemplate.exchange(resourceUrl, HttpMethod.GET, request,
DocumentResponse.class);
String templateId = response.getBody().getDocuments().get(0).get_id();
System.out.println("Template id is : " + templateId);
return templateId;
}
public void sendOutputViaEmail(String outId, String emailId) {
RestTemplate restTemplate = new RestTemplate();
try {
MultiValueMap<String, Object> body = new LinkedMultiValueMap<>();
body.add("outId", outId);
body.add("emailId", emailId);
// set Headers
headers = utils.getHttpHeaders(MediaType.APPLICATION_JSON,
MediaType.MULTIPART_FORM_DATA, utils.apiToken);
// send the request to generate document
HttpEntity requestEntity = new HttpEntity(body, headers);
ResponseEntity<String> generateResponse = restTemplate.postForEntity(urlOutputEmail,
requestEntity, String.class);
System.out.println("Send to edocGen Response : " + generateResponse.getStatusCode());
if (HttpStatus.OK == generateResponse.getStatusCode()) {
System.out.print("Email sent");
}
} catch (Exception e) {
System.out.println("Exception During Sending Email. Check if document id is valid");
}
}
public void getDocsByDatabase(String documentId, String outputFormat, DBParameters dbparmeters) throws Exception {
String outputFileName = UUID.randomUUID().toString();
try {
MultiValueMap<String, Object> body = new LinkedMultiValueMap<>();
body.add("documentId", documentId);
body.add("format", outputFormat);
body.add("outputFileName", outputFileName);
body.add("dbVendor", dbparmeters.getDbVendor());
body.add("dbUrl", dbparmeters.getDbUrl());
body.add("dbLimit", dbparmeters.getDbLimit());
body.add("dbPassword", dbparmeters.getDbPassword());
body.add("dbQuery", dbparmeters.getDbQuery());
// set Headers
headers = utils.getHttpHeaders(MediaType.APPLICATION_JSON,
MediaType.MULTIPART_FORM_DATA, utils.apiToken);
// send the request to generate document
HttpEntity requestEntity = new HttpEntity(body, headers);
ResponseEntity<String> generateResponse = restTemplate.postForEntity(urlBulkGenerate,
requestEntity, String.class);
System.out.println("Send to edocGen Response : " + generateResponse.getStatusCode());
if (HttpStatus.OK == generateResponse.getStatusCode()) {
headers.clear();
headers.set("x-access-token", utils.apiToken);
requestEntity = new HttpEntity(null, headers);
ResponseEntity result = restTemplate.exchange(
baseURL + "output/name/" + outputFileName + "." + outputFormat + ".zip", HttpMethod.GET,
requestEntity, OutputResultDto.class);
processOutput(result, outputFileName, outputFormat, requestEntity, false);
}
} catch (Exception e) {
System.out.println(e.toString());
}
}
private void processOutput(ResponseEntity result, String outputFileName, String outputFormat,
HttpEntity requestEntity , boolean isSingleGeneration) {
OutputDto responseOutput = null;
if ( isSingleGeneration == false) {
outputFormat = outputFormat + ".zip";
}
int retryCounter = 0;
try {
while (result.getBody().getOutput().toString().length() <= 2 && retryCounter < 200) {
System.out.println(
"Output file is still not available. Retrying again!!!! Counter : " + retryCounter);
result = restTemplate.exchange(
baseURL + "output/name/" + outputFileName + "." + outputFormat, HttpMethod.GET,
requestEntity, OutputResultDto.class);
retryCounter++;
}
responseOutput = result.getBody().getOutput().get(0); // naam change kro
} catch (IndexOutOfBoundsException error) {
System.out.println("Error : Output file is not available after 200 tries");
}
System.out.println("Output Document Id generated at edocgen is : " + responseOutput.get_id());
String outputId = responseOutput.get_id();
// output download
headers.clear();
headers.set("x-access-token", utils.apiToken);
headers.setAccept(Collections.singletonList(MediaType.APPLICATION_OCTET_STREAM));
requestEntity = new HttpEntity(null, headers);
ResponseEntity response = restTemplate.exchange(baseURL + "output/download/" + outputId,
HttpMethod.GET, requestEntity, byte[].class);
try {
Files.write(Paths.get(outputFileName + "." + outputFormat), response.getBody());
System.out.println("File downloaded from edocgen with name : " + outputFileName + "." + outputFormat);
} catch (IOException e) {
e.printStackTrace();
System.out.print("Error while Downloading File");
}
}
public void getDocsByApi(String documentId, String outputFormat) throws Exception {
String outputFileName = UUID.randomUUID().toString();
try {
// convert file to byteArrayResource
ByteArrayResource resource = getByteArrayResource();
// create the body request
MultiValueMap<String, Object> body = createBody(resource, documentId, outputFileName, outputFormat);
// set Headers
headers = utils.getHttpHeaders(MediaType.APPLICATION_JSON,
MediaType.MULTIPART_FORM_DATA, utils.apiToken);
// send the request to generate document
HttpEntity requestEntity = new HttpEntity(body, headers);
ResponseEntity<String> generateResponse = restTemplate.postForEntity(urlBulkGenerate,
requestEntity, String.class);
if (HttpStatus.OK == generateResponse.getStatusCode()) {
headers.clear();
headers.set("x-access-token", utils.apiToken);
requestEntity = new HttpEntity(null, headers);
ResponseEntity result = restTemplate.exchange(
baseURL + "output/name/" + outputFileName + "." + outputFormat + ".zip", HttpMethod.GET,
requestEntity, OutputResultDto.class);
System.out.println("Send to edocGen Response : " + generateResponse.getStatusCode());
processOutput(result, outputFileName, outputFormat, requestEntity, false);
} else {
throw new HttpClientErrorException(generateResponse.getStatusCode());
}
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
private MultiValueMap<String, Object> createBody(ByteArrayResource resource,
String documentId, String outputFileName, String outPutFormat) {
MultiValueMap<String, Object> body = new LinkedMultiValueMap<>();
body.add("documentId", documentId);
body.add("inputFile", resource);
body.add("format", outPutFormat);
body.add("outputFileName", outputFileName);
// to download directly the file
body.add("sync", true);
return body;
}
private ByteArrayResource getByteArrayResource() throws IOException {
String fileName = new File(pathFile).getName();
Files.readAllBytes(Paths.get(pathFile));
ByteArrayResource resource = new ByteArrayResource(Files.readAllBytes(Paths.get(pathFile))) {
@Override
public String getFilename() {
return fileName;
}
};
return resource;
}
}
import requests
import uuid
import os.path
import json
edocgen_dev = 'app.edocgen.com'
base_url = "https://%s" %(edocgen_dev)
url = "%s/login" %(base_url)
payload = "{\n\t\"username\":\"[email protected]\",\n\t\"password\": \"password\"\n}"
headers = {
'content-type': "application/json",
'cache-control': "no-cache",
}
response = requests.request("POST", url, data=payload, headers=headers)
x_access_token = response.json()['token']
print ("Using %s for further requests." % x_access_token)
# upload template
url = "%s/api/v1/document" %(base_url)
headers = {
'x-access-token': x_access_token
}
template_file = "/Users/edocgen/Downloads/s1.docx"
files = {'documentFile': open(template_file,'rb')}
response = requests.post(url, files=files, headers=headers)
print(response, response.json())
template_id = response.json()["id"]
print("uploaded document %s with id: %s" %(template_file, template_id))
#Generate request
url = "%s/api/v1/document/generate" %(base_url)
# the output file extension will be added automatically by the edocgen system
outputFileName = "%s.docx" %uuid.uuid4()
generated_format = "pdf"
template_data = "/Users/edocgen/Downloads/s1.json"
inputData = []
with open(template_data, 'r') as content:
inputData = json.load(content)
print(inputData)
# More than one input will always generate the zip file
inputValues = json.dumps({
'sync': True,
'format': generated_format,
'documentId': template_id,
'outputFileName': outputFileName,
'passwordColumn': 'Password',
'markers': inputData
})
outFormat = generated_format
if len(inputData) > 1:
outFormat = 'zip'
headers['content-type'] = 'application/json'
print(url)
response = requests.post(url, data=inputValues, headers=headers, allow_redirects=False)
print(response)
download_to_file = "/Users/edocgen/Downloads/downloaded.%s" %(outFormat)
open(download_to_file, 'wb').write(response.content)
print("File downloaded successfully: %s" % os.path.isfile(download_to_file))
# Delete Template
url = "%s/api/v1/document/%s" %(base_url, template_id)
response = requests.delete(url, headers=headers)
print("Deleted template: %s" %response.json())
# Logout
url = "%s/api/v1/logout" %(base_url)
response = requests.post(url, headers=headers)
print("Logged out of eDocGen: %s" % response.json())
<?php
require "restclient.php";
error_reporting(E_ALL & ~E_NOTICE);
class Edocgen
{
private $api = "";
private $results = "";
private $response = "";
function __construct()
{
// To get user specific token
$ENV["EDOC_USERNAME"] = "[email protected]"; //edocgen username
$ENV["EDOC_PASSWORD"] = ""; //edocgen password
$this->api = new RestClient(["base_url" => "https://app.edocgen.com/"]);
$this->result = $this->api->post("login", [
"username" => $ENV["EDOC_USERNAME"],
"password" => $ENV["EDOC_PASSWORD"],
]);
$this->response = $this->result->decode_response();
# print_r($this->response);
}
public function downloadBulkUsingJson()
{
if ($this->result->info->http_code == 200) {
/*
These variable need to be adjusted as per requirement:
$format = "pdf"; // contains output format
$downloadedFileExt = ".zip"; // download fileout output format. Incase of bulk this will be .zip always
$documentId = "62f063426844520f75344091"; // documentID or TemplateId of document
$MAX_RETRY = 50; //Max number of retries to wait for download. Default 50.
$jsonFilePath = "/Users/Documents/TempWork/project/services/JSON_Data.json"; //path of json file to load
*/
// Start of customizing variables enter here
$format = "pdf";
$downloadedFileExt = ".zip";
$documentId = "62f063426844520f75344091";
$MAX_RETRY = 50;
$jsonFilePath = "/Users/JSON_Data.json";
//End
$curl = curl_init();
$fileName = strtoupper(bin2hex(openssl_random_pseudo_bytes(16)));
$key = $this->response->token;
echo $key . "\n";
// echo $fileName . "\n";
$data = [
"inputFile" => new CURLFILE(
$jsonFilePath
),
"documentId" => $documentId,
"format" => "pdf",
"outputFileName" => $fileName,
];
$inp = [
CURLOPT_URL => "https://app.edocgen.com/api/v1/document/generate/bulk",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => $data,
CURLOPT_HTTPHEADER => ["x-access-token: $key"],
];
curl_setopt_array($curl, $inp);
$response = curl_exec($curl);
curl_close($curl);
echo $response;
if ($response === false) {
print "Could not make successful request\n";
return;
} else {
$response = json_decode($response);
}
$arr = $this->checkOutputGenerated($fileName, $format, $downloadedFileExt);
$retry = 0;
while (
is_null($arr["output"][0]["_id"]) != null &&
$retry < $MAX_RETRY
) {
echo 'Retrying Again.... \n';
echo $retry;
$arr = $this->checkOutputGenerated($fileName, $format, $downloadedFileExt);
$retry++;
}
if (is_null($arr["output"][0]["_id"])) {
echo 'Error !!! Cannot generate output after serveral retries \n';
return "";
} else {
$output_id = $arr["output"][0]["_id"];
$this->downloadOutput($output_id, $fileName, $downloadedFileExt);
}
}
}
public function downloadSingleUsingJson()
{
if ($this->result->info->http_code == 200) {
/*
These variable need to be adjusted as per requirement:
$format = "pdf"; // contains output format
$downloadedFileExt = ".zip"; // download fileout output format. Incase of bulk this will be .zip always
$documentId = "62f063426844520f75344091"; // documentID or TemplateId of document
$MAX_RETRY = 50; //Max number of retries to wait for download. Default 50.
$jsonFilePath = "/Users/Documents/TempWork/project/services/JSON_Data.json"; //path of json file to load
*/
// Start of customizing variables --- enter here
$downloadedFileExt = "pdf";
$documentId = "62f063426844520f75344091";
$MAX_RETRY = 50;
$jsonFilePath = "/Users/JSON_Data_Single.json";
//End
$curl = curl_init();
$fileName = strtoupper(bin2hex(openssl_random_pseudo_bytes(16)));
$key = $this->response->token;
echo $key . "\n";
// echo $fileName . "\n";
$data = [
"inputFile" => new CURLFILE(
$jsonFilePath
),
"documentId" => $documentId,
"format" => "pdf",
"outputFileName" => $fileName,
];
$inp = [
CURLOPT_URL => "https://app.edocgen.com/api/v1/document/generate/bulk",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => $data,
CURLOPT_HTTPHEADER => ["x-access-token: $key"],
];
curl_setopt_array($curl, $inp);
$response = curl_exec($curl);
curl_close($curl);
echo $response;
if ($response === false) {
print "Could not make successful request\n";
return;
} else {
$response = json_decode($response);
}
$arr = $this->checkOutputGenerated($fileName, "", $downloadedFileExt);
$retry = 0;
while (
is_null($arr["output"][0]["_id"]) != null &&
$retry < $MAX_RETRY
) {
echo 'Retrying Again.... \n';
echo $retry;
$arr = $this->checkOutputGenerated($fileName, "", $downloadedFileExt);
$retry++;
}
if (is_null($arr["output"][0]["_id"])) {
echo 'Error !!! Cannot generate output after serveral retries \n';
return "";
} else {
$output_id = $arr["output"][0]["_id"];
$this->downloadOutput($output_id, $fileName, ".".$downloadedFileExt);
}
}
}
public function downloadBulkUsingDB()
{
if ($this->result->info->http_code == 200) {
/*
These variable need to be adjusted as per requirement:
$format = "pdf"; //output format of document generated in edocgen
$dbUrl ="jdbc:mysql://[email protected]:3306/sql6511576/sdtest"; // MySQL DB URL
$dbPassword = "u8M7IYAq7a"; //db password
$dbQuery = "select * from sdtest"; //db query
$dbLimit = "100"; //Number of db entries to be fetched
$zip = ".zip"; //Downloaded file format. bydefault only .zip is supported
$MAX_RETRY = 50; //Max number of tries to download file from edocgen
$documentId = "62f063426844520f75344091"; //Template id from edocgen
*/
// Start of customizing variables
$format = "pdf";
$dbVendor = "mysql";
$dbUrl ="jdbc:mysql://[email protected]:3306/sql6511576/sdtest";
$dbPassword = "u8M7IYAq7a";
$dbQuery = "select * from sdtest";
$dbLimit = "100";
$downloadedFileExt = ".zip";
$MAX_RETRY = 50;
$documentId = "62f063426844520f75344091";
// end of variables
$curl = curl_init();
$fileName = strtoupper(bin2hex(openssl_random_pseudo_bytes(16)));
$key = $this->response->token;
echo $key . "\n";
echo $fileName . "\n";
$data = [
"dbVendor" => $dbVendor,
"dbUrl" => $dbUrl,
"dbLimit" => $dbLimit,
"dbPassword" => $dbPassword,
"dbQuery" => $dbQuery,
"documentId" => $documentId,
"format" => $format,
"outputFileName" => $fileName,
];
$inp = [
CURLOPT_URL => "https://app.edocgen.com/api/v1/document/generate/bulk",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => $data,
CURLOPT_HTTPHEADER => ["x-access-token: $key"],
];
curl_setopt_array($curl, $inp);
$response = curl_exec($curl);
curl_close($curl);
echo $response;
if ($response === false) {
print "Could not make successful request\n";
return;
} else {
$response = json_decode($response);
}
$arr = $this->checkOutputGenerated($fileName, $format, $downloadedFileExt);
$retry = 0;
while (
is_null($arr["output"][0]["_id"]) != null &&
$retry < $MAX_RETRY
) {
echo 'Retrying Again.... \n';
echo $retry;
$arr = $this->checkOutputGenerated($fileName, $format, $downloadedFileExt);
$retry++;
}
if (is_null($arr["output"][0]["_id"])) {
echo 'Error !!! Cannot generate output after serveral retries \n';
return "";
} else {
$output_id = $arr["output"][0]["_id"];
$this->downloadOutput($output_id, $fileName, $downloadedFileExt);
}
}
}
public function sendOutputToEmail($outputId, $emailId)
{
$curl = curl_init();
$key = $this->response->token;
$data = [
"outId" => $outputId,
"emailId" => $emailId,
];
$postdata = json_encode($data);
curl_setopt_array($curl, [
CURLOPT_URL => "https://app.edocgen.com/api/v1/output/email",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => $postdata,
CURLOPT_HTTPHEADER => [
"x-access-token: $key",
"Content-Type: application/json",
],
]);
$response = curl_exec($curl);
curl_close($curl);
print_r($response);
}
//Utility Function to download outputID
public function downloadOutput($outputId, $fileName, $downloadedFileExt)
{
$curl = curl_init();
$key = $this->response->token;
curl_setopt_array($curl, [
CURLOPT_URL => "https://app.edocgen.com/api/v1/output/download/" . $outputId,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => ["x-access-token: $key"],
]);
$raw_file_data = curl_exec($curl);
curl_close($curl);
echo "File Downloaded with name " .
$fileName.
$downloadedFileExt."\n";
file_put_contents(
"./".$fileName.$downloadedFileExt,
$raw_file_data
);
}
public function getTemplateIdviaFileName($fileName)
{
if ($this->result->info->http_code == 200) {
$curl = curl_init();
$key = $this->response->token;
curl_setopt_array($curl, [
CURLOPT_URL =>
"https://app.edocgen.com/api/v1/document/?search_column=filename&search_value=" .
rawurlencode($fileName),
CURLOPT_RETURNTRANSFER => true,
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => ["x-access-token: $key"],
]);
$response = curl_exec($curl);
curl_close($curl);
$arr = json_decode($response, true);
echo "Template ID's with file name - $fileName is : \n";
foreach ($arr["documents"] as $key => $value) {
print_r($value["_id"]);
echo "\n";
}
}
}
//Utility function to check and download output generated in edocGen
public function checkOutputGenerated($fileName, $format, $downloadedFileExt)
{
$curl = curl_init();
$key = $this->response->token;
if ($format == "") {
$uri = $downloadedFileExt;
}
else{
$uri = $format.$downloadedFileExt;
}
echo "Checking Output Generated at: " .
"https://app.edocgen.com/api/v1/output/name/" .
$fileName.
"." .
$uri.
"\n";
curl_setopt_array($curl, [
CURLOPT_URL =>
"https://app.edocgen.com/api/v1/output/name/" .
$fileName.
"." .
$uri,
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "GET",
CURLOPT_HTTPHEADER => ["x-access-token: $key"],
]);
$response = curl_exec($curl);
curl_close($curl);
$arr = json_decode($response, true);
// echo print_r(json_decode($response, true));
// print_r($arr);
return $arr;
}
}
$a = new Edocgen();
//$a->downloadBulkUsingJson();
//$a->downloadSingleUsingJson();
//$a->downloadBulkUsingDB();
//$a->getTemplateIdviaFileName("EDocGen_Testing Invoice.docx");
//$a->sendOutputToEmail("62dac1177b5ea11abd3ba38a", "[email protected]");
?>
let login = require("../edocgen_login");
const fs = require("fs");
const uuid = require("uuid");
const FormData = require("form-data");
let axios = require("axios");
let fileName = uuid.v4();
const headers = {
"Content-Type": "multipart/form-data",
"x-access-token": "null",
};
const hostName = "https://app.edocgen.com/api/v1/document/generate/bulk";
const outputFormat = "pdf";
const documentId = "62bed474447071367d4b1e31"; // Add the template id here
module.exports.generateFiles = function () {
let authToken = login.getToken(function handleUsersList(token) {
headers["x-access-token"] = token;
var formBody = new FormData();
formBody.append("documentId", documentId);
formBody.append("format", outputFormat);
formBody.append("outputFileName", fileName);
formBody.append("inputFile", fs.createReadStream("./JSON_Data.json"));
let config = {
method: "post",
url: hostName,
headers: headers,
data: formBody,
};
console.log(
`https://app.edocgen.com/api/v1/output/name/${fileName}` +
"." +
outputFormat +
".zip"
);
let config_output = {
method: "get",
url:
`https://app.edocgen.com/api/v1/output/name/${fileName}` +
"." +
outputFormat +
".zip",
headers: headers,
};
const MAX_RETRY = 50;
let currentRetry = 0;
function errorHandler() {
if (currentRetry < MAX_RETRY) {
currentRetry++;
console.log(
"Output files are not generated at edocgen yet! Retrying..."
);
sendWithRetry(processResponse);
} else {
console.log("Retried several times but still failed");
}
}
function sendWithRetry(callback) {
axios(config_output)
.then(function (response) {
if (response.data.output.length !== 1) {
throw new axios.Cancel("Operation canceled by the user.");
} else {
callback(response);
}
})
.catch(errorHandler);
}
axios(config)
.then(function (response) {
sendWithRetry(processResponse);
})
.catch(function (error) {
console.log(error);
});
});
};
function processResponse(response) {
const outputId = response.data.output[0]._id;
console.log(
"Edocgen Output Document Generated with Id",
response.data.output[0]._id
);
let config_download = {
method: "get",
url: `https://app.edocgen.com/api/v1/output/download/${outputId}`,
headers: headers,
responseType: "arraybuffer",
accept: "application/zip",
};
axios(config_download)
.then(function (response) {
console.log("Output file is downloaded with " + `${fileName}.zip`);
fs.writeFileSync(`./${fileName}.zip`, response.data);
})
.catch(function (error) {
console.log("Error while downloading");
console.log(error);
});
}
Frequently Asked Questions
Is it possible to create password-protected PDFs with watermarks? Yes, you can generate password-protected PDF files with watermarks. The instructions for opening the password-protected PDF file can be sent to the user via email along with the attachment.
Can I populate a template with multiple data sources? Yes, you can create drafts and update them by populating data from multiple data sources till you generate the desired final output.
Do you support Office365? Yes. You can generate documents with your templates and data files residing in OneDrive.
What are the delivery options available for assembled documents?- Download generated documents. Files are optimized for mobile browser download.
- Email generated files to the respective recipients.
- Sync generated files to AWS S3, OneDrive, or SharePoint DMS.
- Send them for E-Sign.
- Print ready formats PCL5 and PostScript.